Unreal
[Unreal] singleton UGameInstance와 interface의 활용 예시
Lv.Forest
2023. 11. 23. 18:54
1. 게임인스턴스
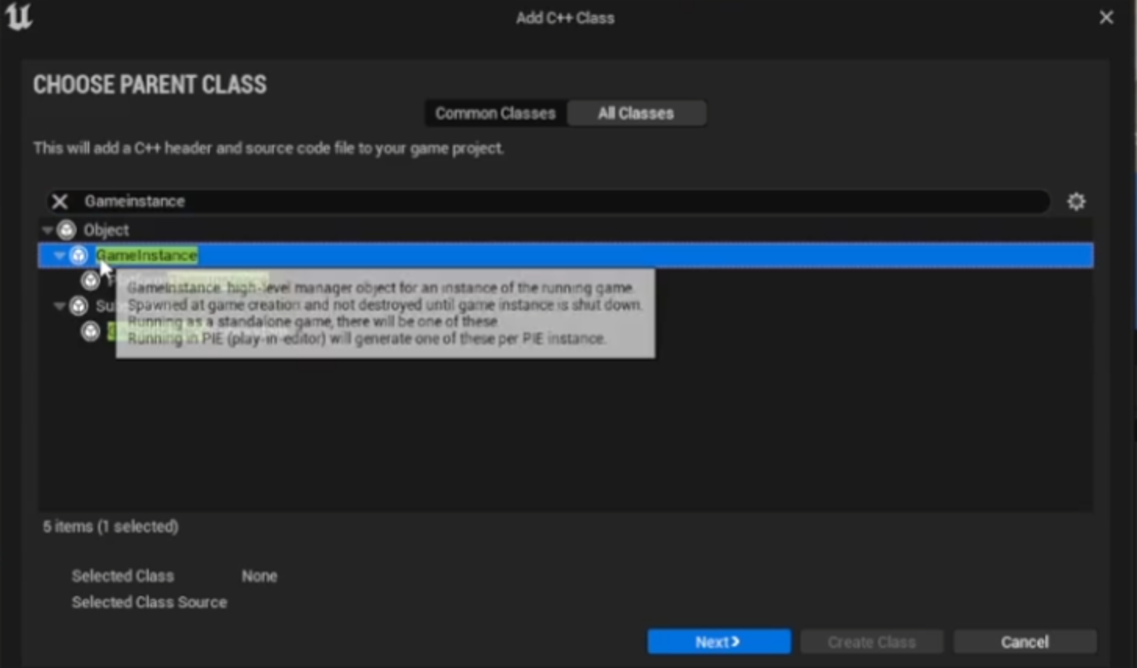
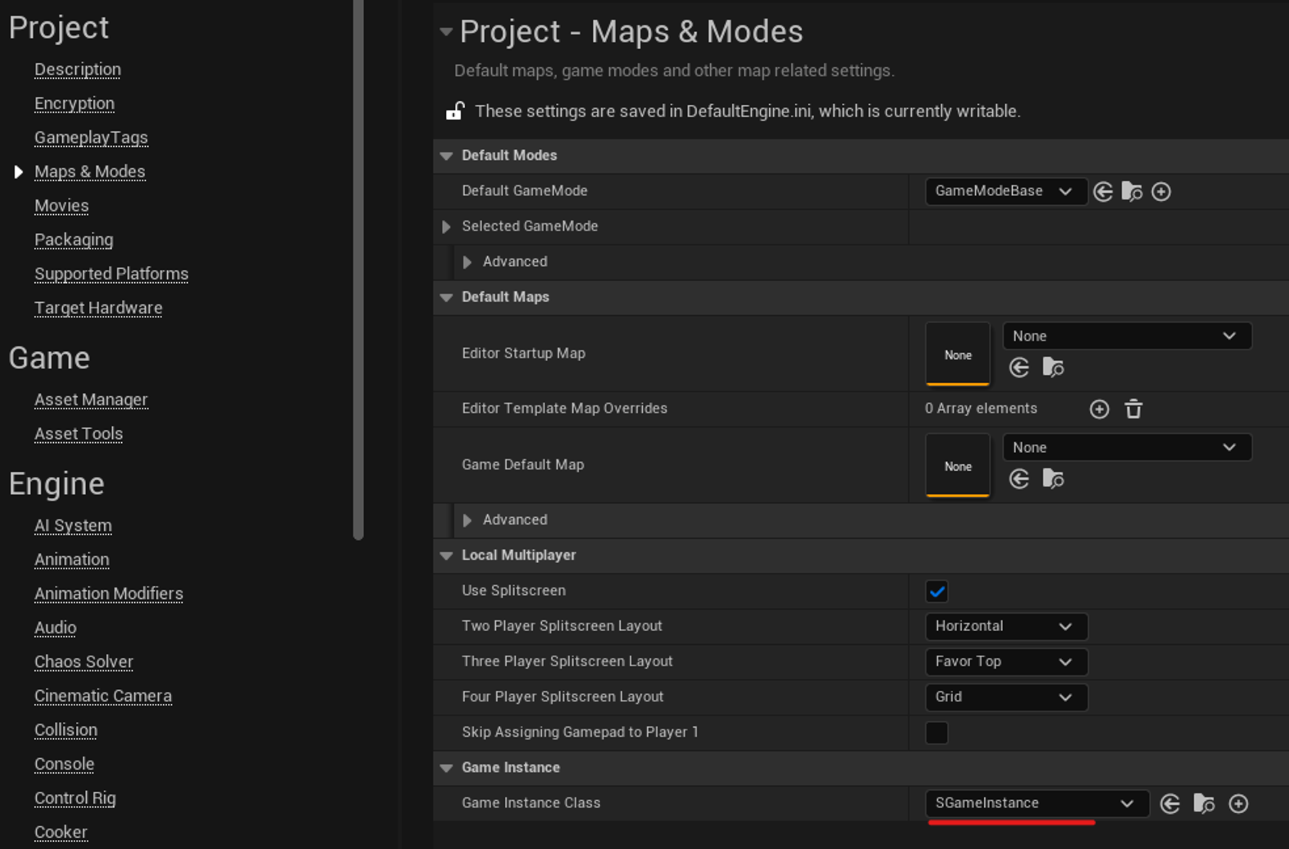
.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "Engine/GameInstance.h"
#include "SGameInstance.generated.h"
/**
*
*/
UCLASS()
class TRACESOFTHEFOX_API USGameInstance : public UGameInstance
{
GENERATED_BODY()
public:
USGameInstance();
virtual void Init() override;
virtual void Shutdown() override;
};
.cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "Game/SGameInstance.h"
#include "Kismet/KismetSystemLibrary.h"
USGameInstance::USGameInstance()
{
}
void USGameInstance::Init()
{
Super::Init();
UE_LOG(LogTemp, Log, TEXT("USGameInstance Init"));
UKismetSystemLibrary::PrintString(this, TEXT("USGameInstance this Init"));
// this 대신 GetWorld 가능
UKismetSystemLibrary::PrintString(GetWorld(), TEXT("USGameInstance GetWorld Init"));
}
void USGameInstance::Shutdown()
{
Super::Shutdown();
UE_LOG(LogTemp, Log, TEXT("USGameInstance Shutdown"));
UKismetSystemLibrary::PrintString(this, TEXT("USGameInstance this Shutdown"));
// this 대신 GetWorld 가능
UKismetSystemLibrary::PrintString(GetWorld(), TEXT("USGameInstance GetWorld Shutdown")); // 월드 객체를 가져올 수 있는 액터들 같은 경우 가능
}
2. 인터페이스
끼워넣을 수 있는 ‘행동’
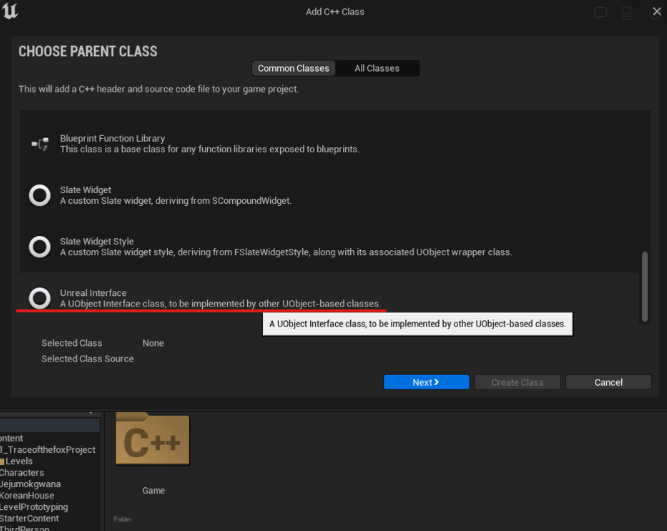
생성해서 만들면 Uclass 와 Iclass가 있다.
.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "UObject/Interface.h"
#include "SFlyable.generated.h"
// This class does not need to be modified.
UINTERFACE(MinimalAPI)
class USFlyable : public UInterface
{
GENERATED_BODY()
};
/**
*
*/
class TRACESOFTHEFOX_API ISFlyable
{
GENERATED_BODY()
// Add interface functions to this class. This is the class that will be inherited to implement this interface.
public:
virtual void Fly() = 0;
// 이렇게 '순수 가상 함수'가 들어있는 클래스를 인터페이스라고 한다.
};
새로 object 클래스를 일단 상속받은 c++ 클래스를 만든다.
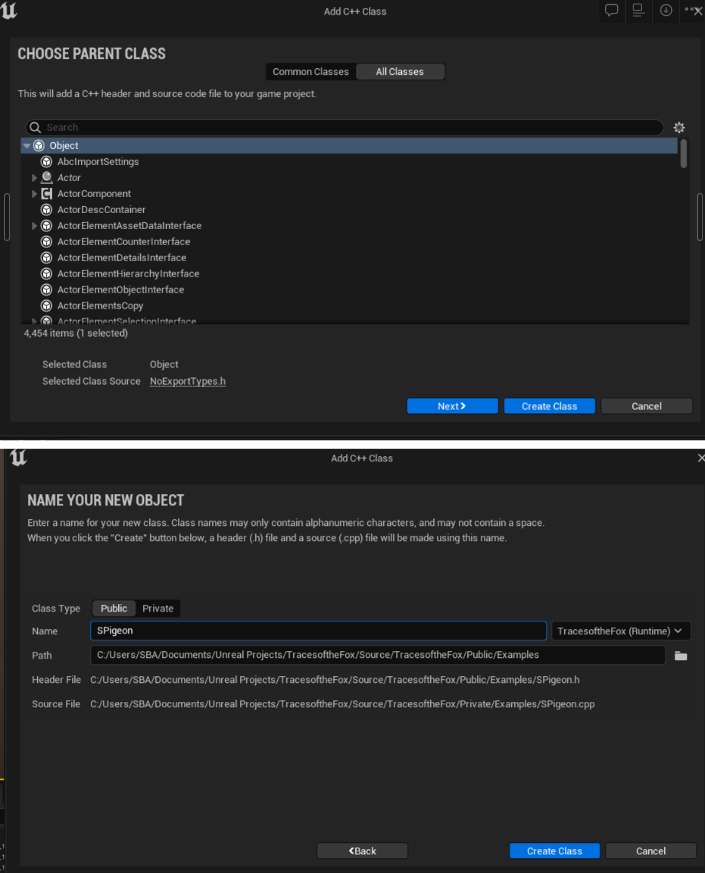
.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "UObject/NoExportTypes.h"
#include "SFlyable.h" // 상속하기 위해 가져옴
#include "SPigeon.generated.h"
/**
*
*/
UCLASS()
class TRACESOFTHEFOX_API USPigeon : public UObject, public ISFlyable // 인터페이스 상속
{
GENERATED_BODY()
public:
USPigeon(); // 생성자
virtual void Fly() override;
private:
UPROPERTY()
FString Name;
};
피죤 cpp
// Fill out your copyright notice in the Description page of Project Settings.
#include "Examples/SPigeon.h"
USPigeon::USPigeon()
{
Name = TEXT("Pigeon");
}
void USPigeon::Fly()
{
UE_LOG(LogTemp, Log, TEXT("%s is now Flying"), *Name)
}
싱글톤에 추가해본다.
// Fill out your copyright notice in the Description page of Project Settings.
#include "Game/SGameInstance.h"
#include "Kismet/KismetSystemLibrary.h"
#include "Examples/SFlyable.h"
#include "Examples/SPigeon.h"
USGameInstance::USGameInstance()
{
}
void USGameInstance::Init()
{
Super::Init();
UE_LOG(LogTemp, Log, TEXT("USGameInstance Init"));
UKismetSystemLibrary::PrintString(this, TEXT("USGameInstance this Init"));
// this 대신 GetWorld
UKismetSystemLibrary::PrintString(GetWorld(), TEXT("USGameInstance GetWorld Init"));
USPigeon* pigeon1 = NewObject<USPigeon>();
ISFlyable* Bird1 = Cast<ISFlyable>(pigeon1);
//현업에서 인터페이스 개념은 대부분 이런 식으로 업캐스팅 하기 위함
if (nullptr != Bird1) {
Bird1->Fly();
}
}
void USGameInstance::Shutdown()
{
Super::Shutdown();
UE_LOG(LogTemp, Log, TEXT("USGameInstance Shutdown"));
UKismetSystemLibrary::PrintString(this, TEXT("USGameInstance this Shutdown"));
// this 대신 GetWorld
UKismetSystemLibrary::PrintString(GetWorld(), TEXT("USGameInstance GetWorld Shutdown")); // 월드 객체를 가져올 수 있는 액터들 같은 경우 가능
}
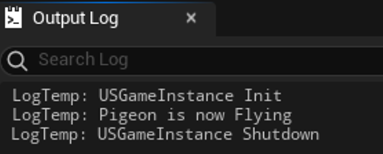